Signed integers in Vyper are a data type allowing storage of positive and negative whole numbers. Here are a few examples:
stored_num: int128
@external
def store_num(num: int128):
self.stored_num = num
@external
def get_num() -> int128:
return self.stored_num
@public
def perform_operations(x: int128, y: int128) -> (int128, int128, int128):
sum = x + y
difference = x - y
product = x * y
return (sum, difference, product)
@public
def compare_values(x: int128, y: int128) -> bool:
return x > y
Vyper implements this fundamental type by offering varying bit lengths, from 8 to 256 bits, for efficient memory and storage management in smart contracts. This article sheds light on the operations possible with signed integers and illustrates their use in Vyper with simple, hands-on examples.
Overview Of Signed Integers
Vyper’s integer classification involves two major types: signed and unsigned integers. The signed integer, specified by the intN keyword (where N varies from 8 to 256, in multiples of 8), allows for negative and positive integer values. This range grants developers flexibility in deciding the appropriate bit length for their specific needs.
Signed integers give us an inclusive range between -2^(N-1) and (2^(N-1) – 1), where N is the bit size of our integer. A crucial note is that integer literals in Vyper do not have a decimal point, indicating they hold whole numbers only. Luckily, unlike Solidity, Vyper has a Decimals data type.
When To Use Signed Integers
Signed integers are pertinent in smart contracts where a value can decrease below zero. Whether it’s a banking contract displaying a negative balance or a gaming contract using negative scores, signed integers encapsulate both positive and negative realm values.
Moreover, signed integers also help in efficient storage utilization, with the freedom to choose from different sizes. Consequently, this assists in more cost-effective smart contract deployments on the Ethereum blockchain.
Bitwise Operators and Bitwise Shifts
Vyper also offers Bitwise operators (&
for bitwise and, |
for bitwise or, ^
for bitwise xor) and Bitwise shifts (<<
for left shift, >>
for right shift) with the signed integers. These advanced operators manipulate the bit levels of the operands, providing intricate control over our data. In particular, Bitwise shifts can only operate with 256-bit wide types.
Example 1: Storing and Retrieving a Signed Integer
In our first example, we store a signed integer in our smart contract and retrieve it with two basic functions
stored_num: int128
@external
def store_num(num: int128):
# Store a signed integer in the contract.
self.stored_num = num
@external
def get_num() -> int128:
# Retrieve the stored signed integer.
return self.stored_num
In this contract, a state variable stored_num
of type int128
is declared. The store_num
function takes in a parameter of type int128
and assigns it to stored_num
. The get_num
method retrieves the stored number when called.
Example 2: Implementing Basic Arithmetic Operations
Next, our example presents a function that performs simple arithmetic operations—addition, subtraction, and multiplication—in a Vyper contract with signed integers.
@public
def perform_operations(x: int128, y: int128) -> (int128, int128, int128):
# Perform basic arithmetic operations with signed integers.
sum = x + y
difference = x - y
product = x * y
return (sum, difference, product)
In this contract, the perform_operations
function has two parameters x
and y
of type int128
. It performs addition (x + y
), subtraction (x - y
), and multiplication (x * y
) on these parameters. It then returns the results as a tuple of int128
values.
Example 3: Comparing Signed Integers
Our last example is a simple comparison operation between two signed integers.
@public
def compare_values(x: int128, y: int128) -> bool:
# Compare two signed integers and return True if x is greater than y.
return x > y
In this contract, the compare_values
function checks if the first parameter x
is greater than the second parameter y
. It takes two int128
integers, compares them using the >
operator, and returns True
if x
is greater than y
.
Best Practices
While using signed integers in Vyper, keep these guidelines in mind:
- Choose Appropriate Bit Length: Select the smallest bit size that suits your requirements to optimize gas usage.
- Avoid Overflow/Underflow: While performing arithmetic operations, be cautious of integer overflows and underflows, as they can lead to unexpected results.
- Be Wary of Zero Division: When using division (
/
), ensure the denominator is not zero to avoid runtime exceptions.
All in all, signed integers serve as a critical data type in Vyper. They allow for a more comprehensive range of values (both positive and negative) and contribute to efficiently building robust smart contracts. As Vyper’s design principles focus on security and simplicity, understanding and effectively using such fundamental data types enhance not only our code’s readability but also its reliability in blockchain ecosystems.
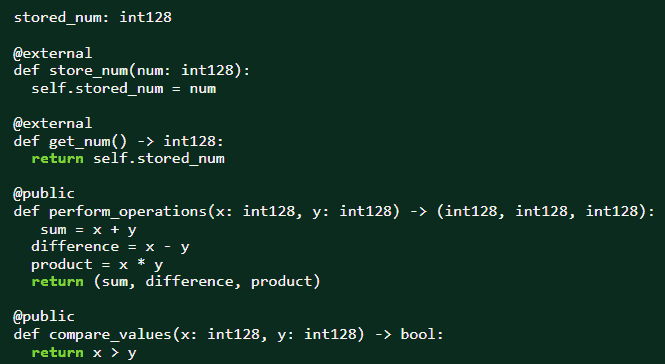