A constructor in solidity is an optional function created with the “constructor” keyword that initializes the state variables of a contract.
A contract can only have one constructor. When a contract is created, the constructor code is executed once and is used to set up the state of the contract during deployment. A constructor can be either public or internal, but it should be noted that an internal constructor marks the contract as abstract. If no constructor is specified, a default constructor is present in the contract.
//SPDX-License-Identifier: MIT
pragma solidity 0.8.13;
contract constructorExample{
string public name;
constructor() {
name = "Tom";
}
function getName() public view returns(string memory){
return name;
}
}
The code above is a very simple contract that uses a constructor as an example. You can see before the constructor is defined, we first create a public string named “name”. Inside the constructor, we reassign the value of this string to “Tom”. When the contract is deployed, the code in the constructor is processed, setting name equal to “Tom”. If you call the “getName” function, you can see the value it returns is “Tom”.
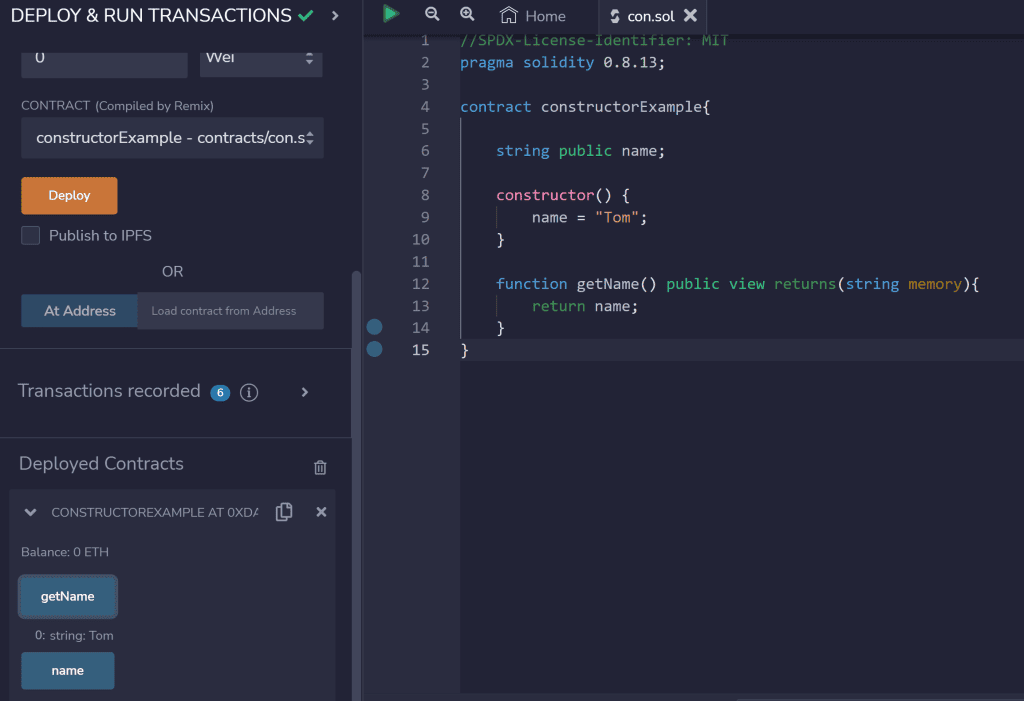
Solidity Constructors provide a host of benefits to developers and users alike.
First, they provide improved security by ensuring that the code is only able to be executed in a secure environment, at the time of deployment. This could prevents malicious code from being executed, as well as any other potential security risks that could be associated with the code. Many developers utilize immutable variables to protect important variables from changing.
Additionally, Solidity Constructors can help speed up development time. They allow developers to quickly create and deploy code without having to manually write each individual line of code. By allowing developers to quickly write and deploy code, it reduces the amount of time needed to build and deploy applications.
Finally, Solidity Constructors can improve efficiency. By having a secure environment in which to execute code, it allows developers to quickly modify and update code without having to rewrite entire sections of code. This reduces the amount of time necessary to develop and maintain applications, leading to improved efficiency.
Constructor Arguments
You can also pass variables through constructors during deployment. This is useful if you want to initialize a variable during deployment and don’t wish to hard-code it into the contract. Many people use this if you have a contract that is deployed many time with different state variable values. Check the example below to learn how to let your constructor accept arguments:
//SPDX-License-Identifier: MIT
pragma solidity 0.8.13;
contract constructorExample{
uint public age;
constructor(uint _age) {
age = _age;
}
function getAge() public view returns(uint){
return age;
}
}
In the code above, you can see we accept an unsigned variable during deployment named “_age”. This value is assigned to the variable “age”, and in this example you can clearly see how there are no numbers in this contract, and that “age” is assigned during creation of what the developer input for the value of “_age”.
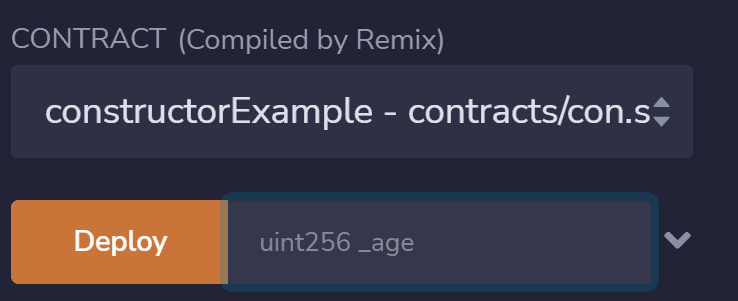
Payable Constructors
You can also make constructors payable. This is useful if you wish to send ETH along with the deployment of a contract. Here is an example of why you may want to make a constructor payable:
//SPDX-License-Identifier: MIT
pragma solidity 0.8.13;
contract payableConstructor {
constructor() payable{
}
function getBackETH() public {
payable(msg.sender).transfer(address(this).balance);
}
}
In this instance, we allow the constructor to receive ETH simply by marking it as “payable”. Then, we have created a very simple function to transfer the entire balance of the contract to whoever calls the function “getBackETH”. This is used for training purposes, and you should know anyone who calls this function will be able to receive the ETH you deposit during deployment.
Constructor Inheritance
The inheritance model of a constructor is very simple, and it reflects many other concepts in inheritance. If you inherit a contract that requires a constructor, you must provide that data during the line you announce it.
Here is an example of a few instances of constructor inheritance:
//SPDX-License-Identifier: MIT
pragma solidity 0.8.13;
contract constructorExample{
uint public age;
constructor(uint _age) {
age = _age;
}
}
contract constructorInheritance is constructorExample(25){
function getAge() public view returns(uint){
return age;
}
}
The above code creates two simple contracts. One contract inherits the constructor of another contract by inputting the information in the main contract declaration line. Below, we show a contract that is more complex and uses the other way of importing constructors:
//SPDX-License-Identifier: MIT
pragma solidity 0.8.13;
contract A {
string public name;
constructor(string memory _name) {
name = _name;
}
}
contract B {
uint public age;
constructor(uint _age) {
age = _age;
}
}
contract C is A, B {
constructor(string memory _name, uint _age) A(_name) B(_age) {
}
function getNameAge() public view returns(string memory, uint){
return (name, age);
}
}
Solidity Constructors are a powerful and necessary tool for anyone building smart contracts, as they offer improved security, faster development time, and improved efficiency of your code. When used correctly, Solidity Constructors can be very useful to any developer, as well to the users of the contract that they are created with.